RESTful API 문서(#2) SpringBoot, Swagger 적용
- WEB / Spring
- 2022. 2. 20. 01:21
1) dependency 등록
2) config 등록
3) controller에 api 작성 annotation 등록으로 가능하다.
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
먼저 pom.xml에 io.spring.fox를 dependency를 등록한다.
@Configuration
public class SwaggerConfig {
@Bean
public Docket api(){
return new Docket(DocumentationType.SWAGGER_2)
.useDefaultResponseMessages(false)
.apiInfo(getApiInfo())
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.ant("/*/**"))
.build();
}
private ApiInfo getApiInfo(){
return new ApiInfoBuilder()
.title("swagger 사용")
.description("swagger를 활용한 API 문서화 자동")
.contact(new Contact("Test Swagger", "http://test.com", "test@gmail.com"))
.version("1.0")
.build();
}
}
@configuration 의 클래스를 만들어 bean을 등록한다. ( config package를 만들어 그 안에 클래스를 만들었다. )
기본적으로 Docket으로 swagger 버전2를 사용한다고 선언 후 리턴한다.
useDefaultResponseMessages(false)로 디폴트 응답 메시지를 사용하지 않겠다고 선언한다.
path의 경우 모든 경로로 설정하였는데, 특정 path로 지정 할 수도 있다.
@ApiOperation(value="사용자 등록", notes="사용자정보 입력하여 사용자 생성")
@ApiImplicitParam(name="id", value="사용자 id"
, required = true
, dataType = "string"
, paramType = "path"
, defaultValue = "None")
@PostMapping("/save")
public Integer saveUser(@RequestBody Long id){
log.info(id);
return homeService.save(id);
}
@ApiOperation(value="sentence 조회", notes="sentence를 조회한다")
@ApiImplicitParams({
@ApiImplicitParam(name="sentenceid",
value="문장 아이디",
required = true,
dataType="int",
paramType="path"),
@ApiImplicitParam(name="title",
value="sentence 제목",
required=true,
dataType="string",
paramType="path")
})
@ApiResponses({
@ApiResponse(code=200, message="성공"),
@ApiResponse(code=404, message="실패")
})
@GetMapping("/sentence")
public String getSentence(int id, String title){
/*Map<String, String > retMap = new HashMap<>();
if(error.hasErrors()){
retMap.put("Status", HttpStatus.NOT_FOUND);
}*/
return homeService.getSentence(id, title);
}
어노테이션 기반으로, api 명세를 한다.
위와 같이 2가지 api 명세를 임의로 만들어 보았다.
@ApiOperation 으로 API에 대한 설명을 작성한다.
@ApiImplicitParams, @ApiImplicitparam 으로 Input에 사용하는 파라미터를 작성할 수 있으며,
@ApiResponse로 응답코드에 대한 메시지를 작성할 수 있다.
작성 후 프로젝트를 Run하면
http://localhost:8080/swagger-ui/index.html
url에서 결과를 확인 ( 3.0.0 버전 url ) 할 수 있다.
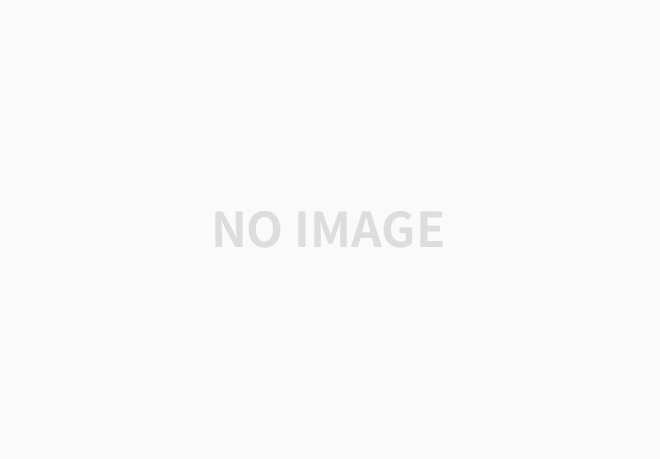
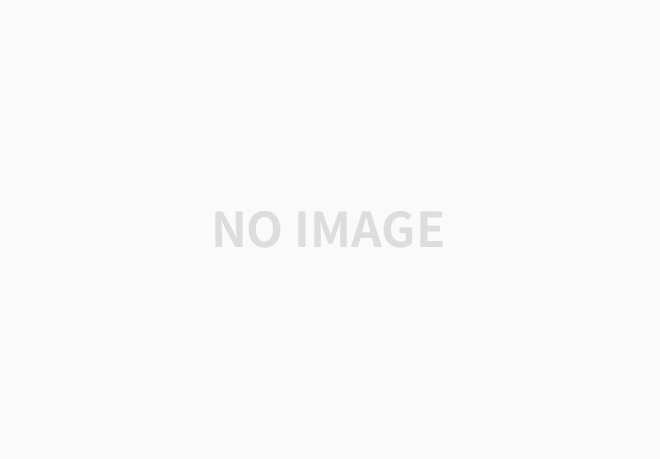
ps. 처음 적용 한 후에, 실행을 하니 에러가 발생하여
확인해보니 Spring 2.6점 대 버전에서는 springfox 3.0.0을 지원하지 않는 것으로 확인되어
Spring을 2.4점 대 버전으로 낮추어 테스트했다 :)
간단히 테스트해보니, 잘만 사용하면 사용성이 매우 높을 것으로 보이나
기본적으로 어노테이션기반으로 작성을 하다보니, 장황하게 설명을 쓰면 API 코드 가독성에 불편함이 생기지 않을까 생각이 들었다.
그리고 spring 코드 안에 어노테이션으로 적용하는 것도 좋지만 Swagger hub를 이용하여 작성하는 게 어찌보면 더 간편하지 않을까? 라는 생각이 들었다.
어찌됐건.. Swagger의 장점은 Try out 기능으로 값을 날려 결과를 리턴해 볼 수 있는 것이니~~ 이 부분에서 만큼은 활용성이 매우 높지 않을까 생각이 든다.
참고)
https://velog.io/@banjjoknim/Swagger
Swagger로 API 문서 자동화를 해보자
Swagger라는 툴을 사용해서 API를 문서화 해보자.
velog.io
https://kim-jong-hyun.tistory.com/49
[Spring] - Swagger 기본사용법 및 API 문서자동화
Spring으로 Rest API를 개발하고 그 API에 대한 문서를 정리하여 해당 API를 사용하는 클라이언트 및 서버 개발자들에게 문서를 정리해서 공유해야하는데 이때 Swagger를 이용하게되면 이런 작업을 보
kim-jong-hyun.tistory.com
'WEB > Spring' 카테고리의 다른 글
[Side Project] MSA 이해하기, 코인 자산 관리 시스템 (0) | 2022.05.23 |
---|---|
[reactive programming] 백엔드 학습, MSA 이해하기 (0) | 2022.04.19 |
[IoC container, 생성자 주입] JAVA > Spring (0) | 2021.12.20 |
웹소켓 사용 법 (0) | 2021.02.10 |
[JUnit] Junit 테스트, @RunWith, @ContextConfiguration 그리고 @SpringApplicationConfiguration (0) | 2021.01.21 |